Currently Empty: $0.00
Blog
Coding Brushup for Java 10: Best Practices & Tips
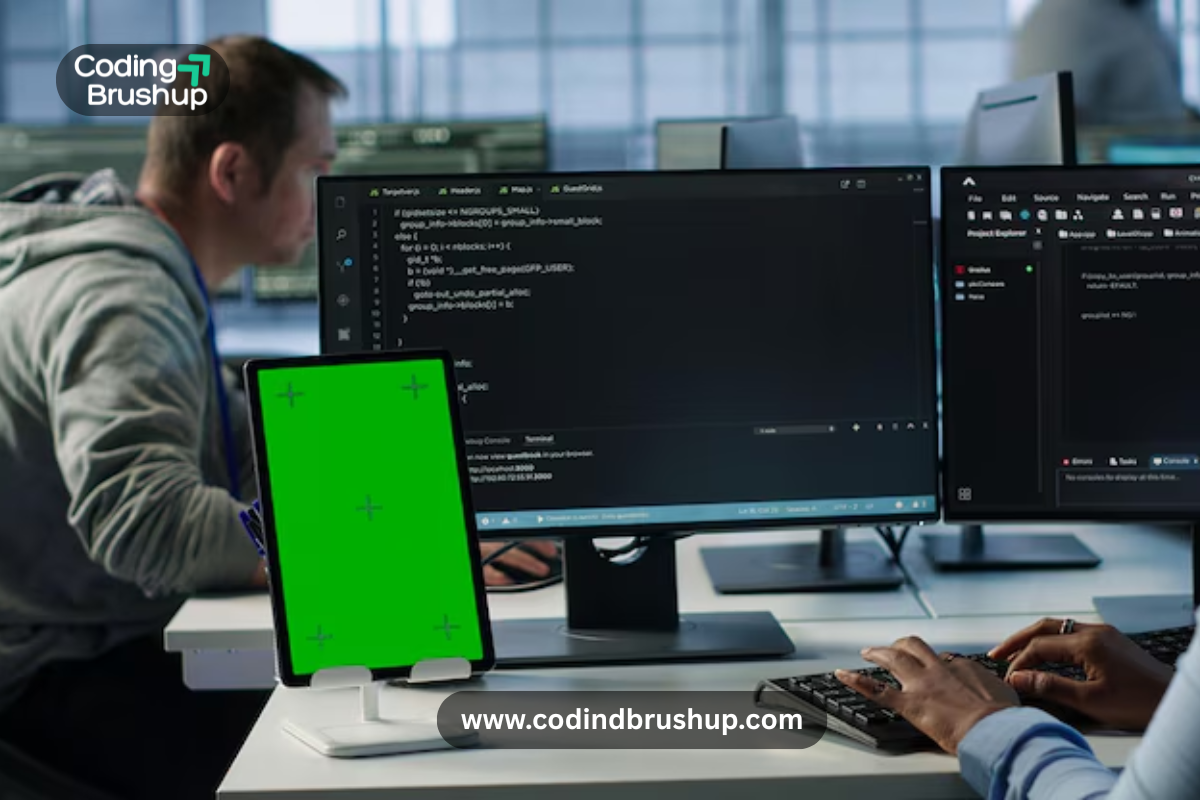
In the fast-evolving world of software development, writing clean, efficient, and maintainable code is paramount. Coding Brushup for Java developers, adhering to best practices not only enhances code quality but also ensures scalability and ease of collaboration. This guide delves into essential Java coding best practices, offering actionable insights to elevate your programming standards.
1. Embrace Meaningful Naming Conventions
Clear and descriptive names for classes, methods, and variables significantly improve code readability and maintainability. Avoid ambiguous names like temp
or data
; instead, opt for names that convey purpose, such as calculate Total Price
or user Age
. Consistent naming conventions also facilitate smoother collaboration among developers.
2. Adhere to the DRY Principle (Don’t Repeat Yourself)
Repetition in code leads to redundancy and increases the risk of errors. By creating reusable methods or classes, you can eliminate duplicate code, making your codebase more concise and easier to maintain. For instance, instead of duplicating validation logic across multiple methods, centralize it in a single utility class.
3. Implement the Single Responsibility Principle (SRP)
Each class should have one reason to change, meaning it should only have one job or responsibility. By ensuring that classes are focused on a single task, you enhance code clarity and make it easier to modify or extend functionality without unintended side effects.
4. Utilize Exception Handling Wisely
Proper exception handling is crucial for building robust applications. Avoid using exceptions for control flow; instead, catch specific exceptions and handle them appropriately. Employ try-with-resources statements to manage resources like file streams, ensuring they are closed automatically.
5. Avoid Hardcoding Values
Hardcoding values, such as file paths or configuration settings, can lead to inflexible code. Instead, use constants or external configuration files to store such values, making your application more adaptable to changes and easier to maintain.
6. Leverage Coding Brushup forJava Streams and Lambda Expressions
Introduced in Java 8, Streams and Lambda expressions allow for more concise and readable code, especially when dealing with collections. They enable functional-style operations like filtering, mapping, and reducing, which can simplify complex data processing tasks.
7. Prioritize Code Readability
Code is often read more frequently than it’s written. Therefore, prioritize readability by using consistent indentation, meaningful comments, and clear logic. Avoid deep nesting and complex conditionals; instead, break down complex methods into smaller, more manageable ones.
8. Follow the SOLID Principles
The SOLID principles are a set of five design principles that promote object-oriented design and programming:
- Single Responsibility Principle (SRP): A class should have one, and only one, reason to change.CodeJava+5JavaDZone+5perfectelearning.com+5
- Open/Closed Principle (OCP): Software entities should be open for extension but closed for modification.JavaDZone+1perfectelearning.com+1
- Liskov Substitution Principle (LSP): Objects of a superclass should be replaceable with objects of its subclasses without affecting the correctness of the program.
- Interface Segregation Principle (ISP): No client should be forced to depend on methods it does not use.JavaDZone
- Dependency Inversion Principle (DIP): High-level modules should not depend on low-level modules. Both should depend on abstractions.
Adhering to these principles leads to more modular, flexible, and maintainable code. JavaDZone
9. Optimize Performance Thoughtfully
While it’s important to write efficient code, premature optimization can lead to unnecessary complexity. Focus on writing clear and correct code first; then, profile and optimize performance-critical sections as needed. Utilize tools like JProfiler or Visual VM to identify bottlenecks in your application.
Conclusion
By integrating these best practices into your Coding Brushup for Java development workflow, you can produce code that is clean, efficient, and maintainable. Remember, the goal is not just to write code that works, but to write code that is easy to understand, modify, and extend. Continuous learning and adherence to these principles will set you on the path to becoming a proficient Java developer.